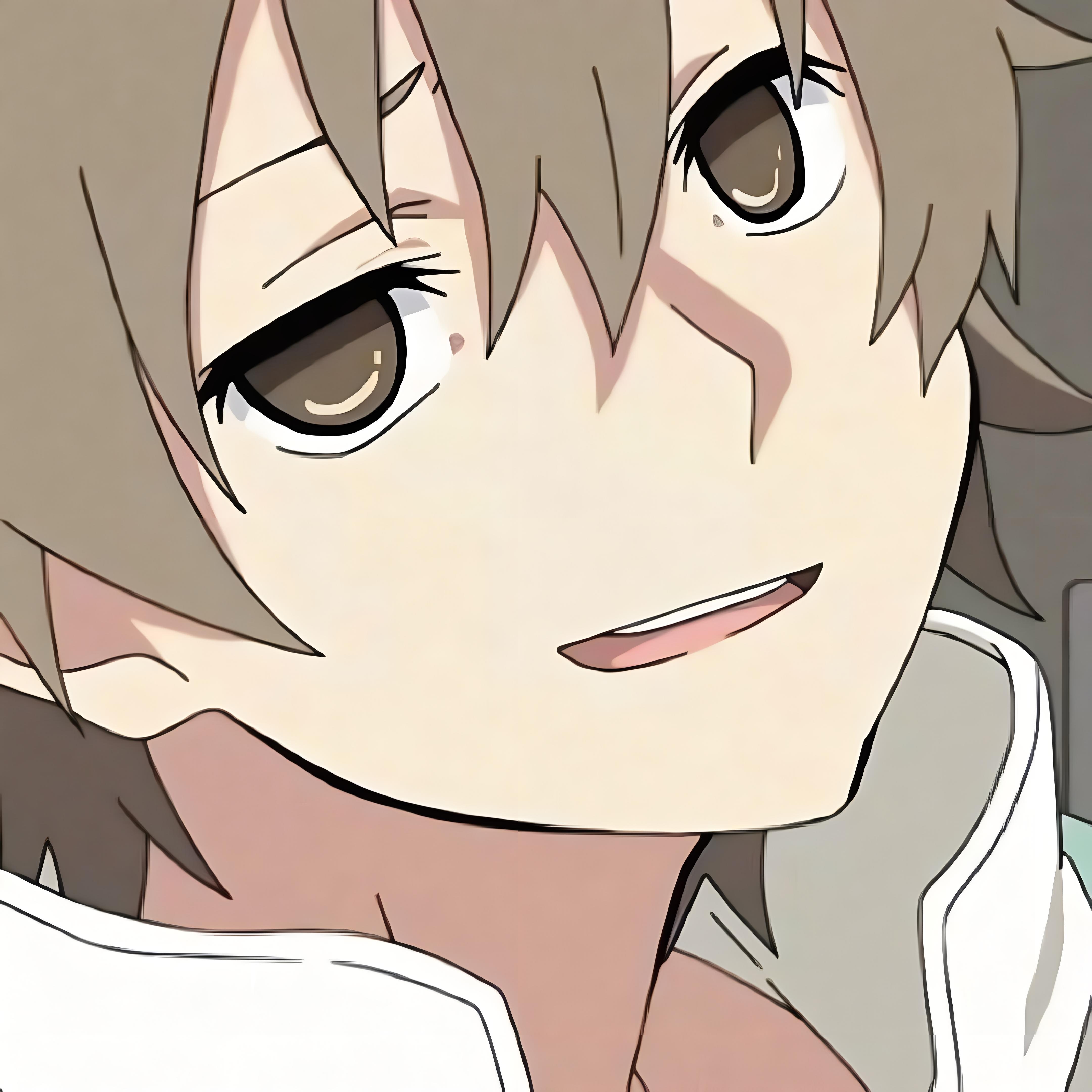
一介闲人
一介闲人
请求的链路:
Request -> Tomcat -> Filter(过滤器) -> Servlet -> Interceptor(拦截器) -> Controller
请求响应链路,与请求相反:
Controller -> Interceptor(拦截器) -> Servlet -> Filter(过滤器) -> Tomcat -> Response
实现通用的功能过滤,如敏感词过滤、字符集编码设置、响应数据压缩等。
主要用于实现项目的业务判断,如日志记录,包括请求的 URL、参数、客户端 IP 等信息。记录控制器方法的执行时间,用于性能监控。对响应进行统一的格式处理,如添加特定的 JSONP 回调函数等。
1、拦截器
** 拦截器类 ** :实现Filter,重写init、destroy、doFilter这三个方法
import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
import java.io.IOException;
@WebFilter(urlPatterns = "/*")
public class TestFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
System.out.println("过滤器Filter初始化");
}
@Override
public void destroy() {
System.out.println("过滤器Filter销毁");
}
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
System.out.println("过滤器Filter开始执行doFilter");
filterChain.doFilter(servletRequest,servletResponse); //请求放行
System.out.println("过滤器Filter结束执行doFilter");
}
}
** 启动类 **:加 @ServletComponentScan 注解
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletComponentScan;
@SpringBootApplication
@ServletComponentScan
public class SpringbootInterceptorApplication8095 {
public static void main(String[] args) {
SpringApplication.run(SpringbootInterceptorApplication8095.class, args);
}
}
2、过滤器
** 过滤器类 **:实现HandlerInterceptor,重写preHandle、postHandle、afterCompletion这三个方法
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.HandlerInterceptor;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@Component
public class LoginInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
System.out.println("拦截器执行了preHandle");
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
System.out.println("拦截器执行了postHandle");
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
System.out.println("拦截器执行了afterCompletion");
}
}
** 配置类 **: 将过滤器注入Spring容器
import com.lilke0.springbootinterceptor.interceptor.LoginInterceptor;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import javax.annotation.Resource;
@Configuration
public class InterceptorConfig implements WebMvcConfigurer {
//注入拦截器
@Resource
private LoginInterceptor loginInterceptor;
@Override
public void addInterceptors(InterceptorRegistry registry) {
//注册拦截器
registry.addInterceptor(loginInterceptor)
//添加需要拦截的路径
.addPathPatterns("/**");
}
}
或者, 另一种注入方式
import com.lilke0.springbootinterceptor.interceptor.LoginInterceptor;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@SpringBootApplication
public class SpringbootInterceptorApplication8095 {
public static void main(String[] args) {
SpringApplication.run(SpringbootInterceptorApplication8095.class, args);
}
@Bean
public WebMvcConfigurer configurer() {
return new WebMvcConfigurer() {
@Override
public void addInterceptors(InterceptorRegistry registry) {
//启用拦截器
registry.addInterceptor(new LoginInterceptor()).addPathPatterns("/admin/**")
.excludePathPatterns("/admin/login")
.excludePathPatterns("/admin/login_check");
}
};
}
}
评论